Vue.js + Nuxt with Docker
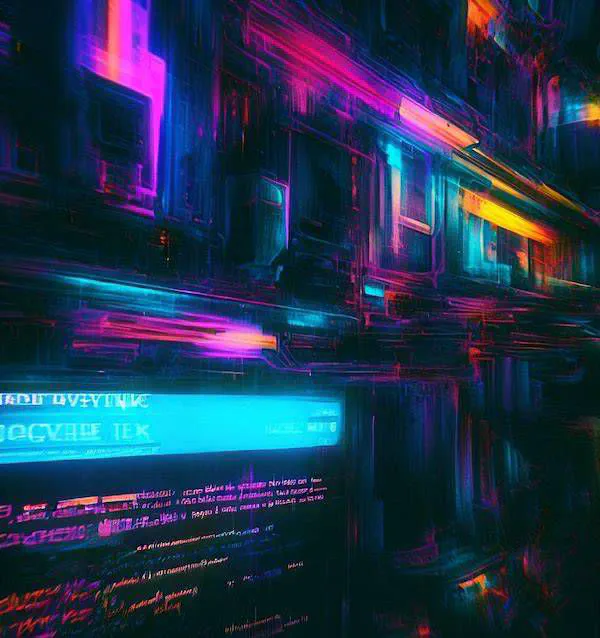
Vue.js and Nuxt are powerful frameworks for building web applications. Docker is a containerization platform that can be used to package and deploy these applications.
To dockerize a Nuxt application, a Dockerfile is created that specifies the steps required to build the image. This typically includes installing Nuxt and the application’s dependencies.
FROM node:20-alpine
RUN mkdir -p /usr/src/nuxt-app
WORKDIR /usr/src/nuxt-app
COPY . .
RUN npm ci && npm cache clean --force
RUN npm run build
ENV NUXT_HOST=0.0.0.0
ENV NUXT_PORT=3000
EXPOSE 3000
ENTRYPOINT ["node", ".output/server/index.mjs"]
Once the Dockerfile is created, the image can be built using the docker build
command. The image can then be run using the docker run
command.
Dockerizing web applications offers a number of benefits, including:
- Ease of deployment: Docker images can be deployed to any environment that has Docker installed.
- Security: Docker images are isolated from the host environment, which can improve security.
- Reproducibility: Docker images can be used to reproduce an application’s environment, which can be helpful for debugging and testing.
If you would like to serve the above application on a website e.g. https://example.com with a free LetsEncrypt certificate, consider using nginxproxy/acme-companion and edit your docker-compose.yml
to include the web app with this snippet:
web-app:
environment:
- LETSENCRYPT_HOST=example.com,www.example.com
- VIRTUAL_HOST=example.com,www.example.com
- VIRTUAL_PORT=3000
expose:
- '80'
build: /home/user/nuxt-website/
networks:
- nginx-proxy
restart: unless-stopped
Bonus - systemd
Systemd is a system and service manager for Linux operating systems.
Another option - if you don’t have Docker - would be to create a custom systemd service, create a new file in /etc/systemd/system/nuxt.service
like this, and edit it to fit your needs:
[Unit]
After=network.target
Description=run Nuxt application
[Service]
ExecStart=env PATH=$PATH:/home/user/.nvm/versions/node/v20.5.0/bin node .output/server/index.mjs
WorkingDirectory=/home/user/nuxt-website
User=user
Group=user
[Install]
WantedBy=multi-user.target
You just got your systemd compatible service, for more information check the ArchWiki
# enable the service (to start on boot)
sudo systemctl enable nuxt.service
# start it
sudo systemctl start nuxt.service
# stop it
sudo systemctl stop nuxt.service
# disable it
sudo systemctl disable nuxt.service
# check the status
systemctl status nuxt.service
# check the logs
journalctl -u nuxt
You can now dockerize your Nuxt app and serve it on a website with a free LetsEncrypt certificate OR with systemd 😉